The Set object lets you store unique values of any type, whether primitive values(strings, numbers or booleans) or object references.
Creating a set:
There are 2 ways to create a set, which are –
1. An Empty Set
An empty set does not has any value and after creation we need to add values using add() method.
const mySet = new Set()
2. A set with some values
If we want to initialize a set with some pre-defined value and then just pass an iterable to it as shown below –
const mySet = new Set([1,2,3,4,5,6,7,8,9]);
Adding an element to a Set:
We can add elements to a set using add() method provided by the Set class.
You can see above that we tried to add 5 twice but set just added it once, as said in the definition above, set stores unique elements only.
Deleting element from a Set:
1. Deleting a single element from the Set
To delete any specific element from a set, just call delete() and pass the element to be deleted as param.
2. Deleting all elements from the Set
To delete all the elements of a set we can just use the clear() method
Finding size of a Set:
To find the size of a set we can use size property of Set class.
mySet.size
Check existence of a element in Set:
To check whether an element exists in the Set, we use has() method of Set class.
mySet.has(1) //true
mySet.has(25) //false
Iterating a Set:
There are many ways to iterate over the elements of a set –
- using for of loop
- using forEach loop
- using array conversion
- using keys() methods
- using values() method
- using entries() method
In Set you observe that key and value of each element is same hence keys() and values() gives you same array of elements for a Set.
Mergin multiple Set:
To merge two or more sets we can use spread operator as shown below.
var merged = new Set([...set1, ...set2, ...set3])
Converting Set to Array:
1. Using Array.from() method
let myArr = Array.from(mySet);
2. Using spread operator
let myArr = [...mySet];
Operations of Set
1. Subset

a set A is a subset of a set B, or equivalently B is a superset of A, if A is “contained” inside B, that is, all elements of A are also elements of B.
2. Union
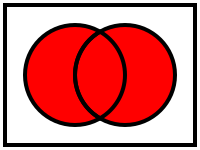
The union of two sets A and B is the set of elements which are in A, in B, or in both A and B
3. Intersection

the intersection of two sets A and B is the set that contains all elements of A that also belong to B (or equivalently, all elements of B that also belong to A), but no other elements.
4. Difference

The difference of set A from setB, denoted by B-A, is the set of all the elements of set B that are not in set A.
Let me know your thoughts